Sum of n Natural Numbers in C
Here you will learn about the natural numbers and get the program code to calculate Sum of n Natural Numbers in c programming. We will create this program by using 5 different ways with loops, functions, and recursion in C Programming.
What is Natural Number?
A natural number is any positive integer, or whole number, greater than zero. for examples :- 1, 2, 3, 4, 5, 6…… and so on.
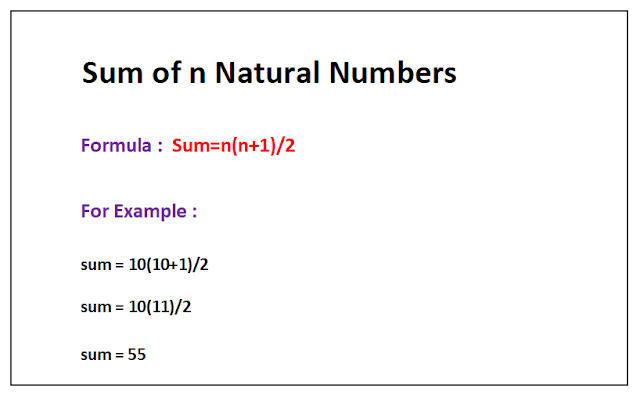
Sum of n Natural Numbers Formula?
sum = n(n+1)/2
where n is number of terms
For example:
n=10;
sum = 10(10+1)/2
sum = 10(11)/2
sum = 55
Different 5 Ways to find Sum of n Natural Numbers in C
Here, we will do this program using 5 different ways like using while loop, do-while loop, for loop, functions, and recursion.
Using while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | // Sum of n Natural Numbers in c using while loops #include <stdio.h> int main() { int i , s = 0; int n = 10; i= 1; while (i <= n) { s += i; i++; } printf("\nSum = %d", s); return 0; } |
Output

Using do while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | //Sum of n Natural Numbers in c using Do-while loops #include <stdio.h> int main() { int i , s = 0; int n = 10; i= 1; do{ s += i; i++; }while(i<=n); printf("Sum = %d", s); return 0; } |
Output

Using for loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | // Sum of n Natural Numbers in c using for loop #include <stdio.h> int main() { int i , s = 0; int n = 10; for(i=0; i<=n;i++) { s += i; } printf("Sum = %d", s); return 0; } |
Output

Using functions
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | // Sum of n Natural Numbers in c using functions #include <stdio.h> int sum_of_natural_numbers(int n) { int i , s = 0; for(i=0; i<=n;i++) { s += i; } return(s); } int main() { int i , s = 0; int n = 10; s=sum_of_natural_numbers(n); printf("Sum is = %d", s); return 0; } |
Output

Using recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | // Sum of Natural Numbers using recursion #include <stdio.h> int sum_of_natural_numbers(int n) { if (n != 0) return n + sum_of_natural_numbers(n - 1); else return n; } int main() { int num = 10; printf("\nSum = %d", sum_of_natural_numbers(num)); return 0; } |
Output

Check out our other C programming Examples