Array in C Programming
Here you will learn the basic tutorial of array in c programming with examples.
What is Array in C?
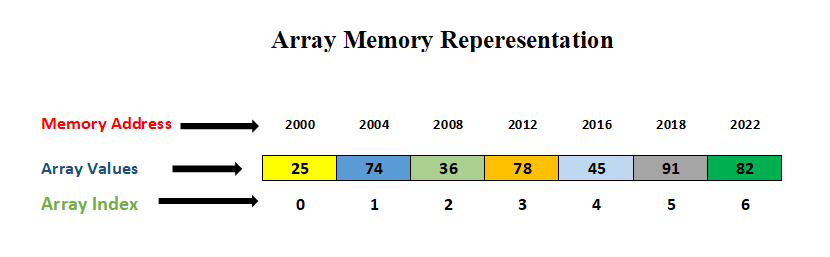
Advantages and Disadvantages of Array in C
Advantage of Array | Disadvantage of Array |
Code Optimize : write Less code to the access the data. | Array Size Fixed : Array size is static(fixed), The memory allocated to array cannot be changed(increased or decreased) after declaration. It is not flexible as link list in Data Structure. |
Ease of traversing : using the loop(for, while, do-while) we can access the elements of an array quickly and easily. | |
Ease of sorting : need few lines code, to sort array elements easily. | |
Random Access : we can access any random element quickly using index number. |
One Dimensional Array in C
A one-dimensional array or 1d array C is a linear data structure that stores a fixed-size sequential collection of elements of the same data type. It is also known as a single-dimensional array. It is declared by specifying the array type followed by the array name and size of the array enclosed in square brackets.
Syntax:
data_type array_name[size];
Example:
int arr[10];
Declaration of Array in C
Example: int arr[7];
Initialization of Array in C
Initialization of the array elements in C is an easy way, by using the index of every element. Initialize every element by using the index.
arr[1]=10;
arr[2]=15;
arr[3]=20;
arr[4]=25;
arr[5]=30;
arr[6]=35;

Example: Initialization of Array
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | //Initialization of Array in C #include<stdio.h> int main() { int i,score[5]; // array declaration score[0]=95; //array initialization score[1]=79; //array initialization score[2]=82; //array initialization score[3]=73; //array initialization score[4]=86; //array initialization // print the elements of array score for(i=0;i<5;i++) { printf("\n%d",score[i]); } return 0; } |
Declaration with Initialization Array in C
In c, we can initialize the array at the declaration time. Let’s see the following code.
int score[5]={10,20,30,40,50};
in this condition, no need to assign size of array, it can also be written as
int score[]={10,20,30,40,50};
Example: Declaration with Initialization
1 2 3 4 5 6 7 8 9 10 11 12 13 | //Declaration with Initialization Array in C #include<stdio.h> int main() { int i,score[5]={95,79,82,73,86}; // array declaration and initialization // print the elements of array score for(i=0;i<5;i++) { printf("\n%d",score[i]); } return 0; } |
Output
One Dimensional Array Example with code
Here, I am writing some programs, so that your all concept related to single dimension array would be clear. if still have any problem then write a comment at the last of the article. I will try to resolve with my best.
1. C Program to Sort an Array in Ascending Order
2. C Program to Find Largest Array Element
3. C program to Find Sum and Average of an Array Elements
4. C Program to Search Element in an Array
5. C Program to Insert an Element in an Array
1d Array Assignment work
Make the following programs to test your knowledge and send code to me in the comment box. If I like, I will publish your code on my coderevise.com with your reference.
1. C program to sort an array in descending order.
2. C program to find duplicates in a list.
3. C program to find second largest number in an array.
4. C program to merge elements of two arrays in third one.
Two Dimensional Array in C
What is Two Dimensional Array in C ?
The two-dimensional array is defined in Matrix format, in that rows and columns play an active role. 2D arrays are used to store the bulk amount of data at once, which can be processed in functions.
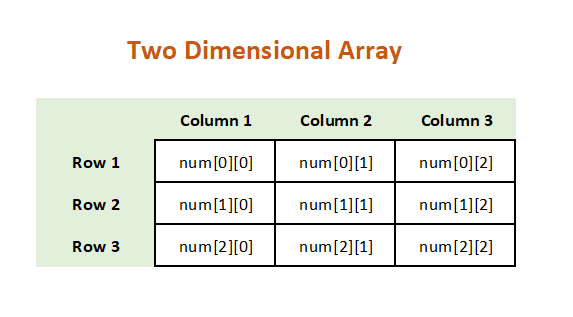
Advantages and Disadvantages of 2D Array in C
2D Array Advantages | 2D Array Disadvantages |
Ease to access : element can be access easily by index number. | Array Size Fixed : Array size is static(fixed), The memory allocated to array cannot be changed(increased or decreased) after declaration. |
Represent matrix : Two Dimensional array is used to represent matrix. | |
Store multiple values : It can be used to store multiple values and work with efficiently. |
Declaration of 2D Array in C
We can declare the Two Dimensional Array in C as the following :
Syntax : data_type array_name[number of rows][number of columns];
Example : int num[3][3];
Initialization of Two 2D Array in C
2D Array initialization can be done at the declaration time of 2D array. Here are some examples of initializing a 2d array :
Method 1
int num[3][3] = {{5,9,7},{3,9,8},{12,20,6}};
Method 2
Using for Loop :
for(int j=0 ;j < 3 ;j++)
scanf(“%d”,&num[i][j]);
}
}
Two dimensional array example in C
#2. Write a C program to Add Two Matrix using Two dimensional Array.
#3. Write a C Program to Multiply Two Matrices using Two dimensional Arrays.
#4. Write a C program to Transpose a Matrix in C.
Difference between 1D Array and 2D Array
2d Array Assignment work
1. C program to create a mirror image of two dimensional array
for example:-
2. C program to arrange row elements in ascending order.
3. C program to check matrix is a sparse matrix or not
Check out our other C programming Examples