Loops in C with Examples
Here you will learn the different Loops in C with Examples. In this tutorial, we will explain all the different types of loops with details and examples in C programming language.
What are the loops in C
Loop is a technique that is used to repeat the set of instructions multiple times until a certain condition is met.
How many loops are there in C?
There are three basic types of loops available in C programming.
1. for loop
2. while loop
3. do-while loop
For Loop in C
The for loop is the most commonly used loop in C. It is used to execute a set of statements a certain number of times. The syntax of the for loop is:
Syntax
1 2 3 4 5 6 | for (initialization; condition; increment/decrement) { // Statements } |
The initialization statement is used to set the initial value of the loop variable.
The condition statement is used to check for the loop termination condition.
The increment/decrement statement is used to update the loop variable.
for loop example in C
1 2 3 4 5 6 7 8 9 10 11 | //Print 1 to 10 numbers using for loop #include<stdio.h> void main() { int i; printf("\n\nprint 1 to 10 numbers using for loop"); for(i=1;i<=10;i++) { printf("\n%d",i); } } |
Output
while Loops in C
The while loops in c is used to execute a set of statements until a certain condition is met. The syntax of the while loop is:
Syntax
1 2 3 4 5 6 7 8 | while (condition) { // Statements //increment or decrement } |
while loop example in C
1 2 3 4 5 6 7 8 9 10 11 12 | //Print 1 to 10 numbers using While Loop #include<stdio.h> void main() { int i=1; printf("\n\nprint 1 to 10 numbers using while loop"); while(i<=10) { printf("\n%d",i); i++; } } |

do while Loops in C
The do while loop is similar to the while loop but differs in that the loop body is always executed at least once. The syntax of the do–while loop is:
Syntax
1 2 3 4 5 6 7 | do { // Statements // increment/ decrement } while (condition); |
do while loop example in C
1 2 3 4 5 6 7 8 9 10 11 12 | //Print 1 to 10 numbers using do-while Loop #include<stdio.h> void main() { int i=1; printf("\n\nprint 1 to 10 numbers using do-while loop"); do { printf("\n%d",i); i++; }while(i<=10); } |
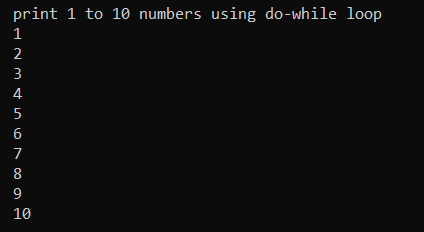
Difference between while and do while loops in C
The differences are given in the following table :-
while loop | do while loop |
⮞ The main difference between while and do-while loops is that while loops test the condition before executing the loop body. | ⮞ do-while loops execute the loop body once before testing the condition. |
⮞ while loop will execute its body as long as the condition remains true, | ⮞ do-while loop will execute its body at least once, and then keep executing as long as the condition remains true. |